Introduction
The Beacon Classic web apps allow you to add some of your own customizations. For example, you can create a JavaScript plugin to listen for web page events and process custom code based on your requirements. For details, see the OTT Plugins documents.
Beacon page events are triggered by user interactions with the Beacon Classic app. This diagram shows the relationship between the user, your Beacon Classic web app and your plugin code.
Use cases
Here are some use cases when listening to page events:
onBeaconPageLoad
- When this event is triggered, you can add custom buttons, populate custom sections, and create custom pages for your Beacon Classic web apploadedBeaconVideoMetadata
- When this event is triggered, you can display data for the current video in your Beacon Classic web app
Event listeners
The following page events can be listened for by your OTT plugin code:
Event | Description |
---|---|
beforeBeaconPageLoad |
Called before the page starts to load all of the required elements. This includes the API calls to fetch asset and playlist details |
onBeaconPageLoad |
Called when all of the required elements to render the page are available and all checks are complete (entitlements, episodes list, etc) |
onBeaconPageChange |
Called when there is a change on the Beacon Classic web app page. For example, when you are watching a video on the details page, and select a new series, season or episode. For the Live TV page, it is also called when the selected linear channel changes. |
loadedBeaconVideoMetadata |
Called when the metadata for the current video has been loaded |
onBeaconPageUnload |
Called after the destroy() from the superclass finishes |
Event data
When listening to page events, you can display the event data which is available for use in your custom code.
Data element | Type | Description |
---|---|---|
page_type |
enum | A unique page type for the current page being viewed. Values:
|
slug |
string | A unique id for your custom page |
asset_id |
integer | Sent in the event object for these pages:
|
playlist_id |
integer | Sent in the event object for the playlist_view_all page
|
page_id |
integer | Sent in the event object for the home page
|
bc_account_id |
integer | Sent in the loadedBeaconVideoMetadata event |
device |
string | 'web' |
video_id |
integer | Sent in the loadedBeaconVideoMetadata event |
user_language |
string | The language code for the current language from the Beacon API |
entitlements |
boolean | Displays whether the content is free or if the user is entitled to see the content |
Examples
Here are some examples of actual page event data:
beforeBeaconPageLoad
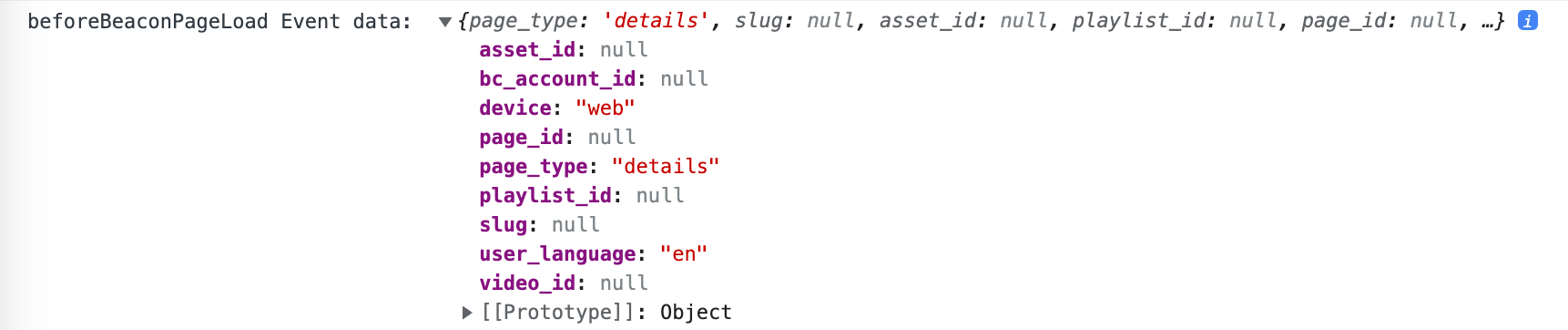
onBeaconPageLoad

loadedBeaconVideoMetadata

onBeaconPageChange

onBeaconPageUnload

Module implementation
The following shows how to listen to Beacon Classic web page events. For some customization examples, see the OTT Plugins documents.
While our examples use JavaScript modules, you may implement your JavaScript in the manner of your choice. Further details on this module implementation can be found in the Implementing OTT Plugin Code Using Modules document.
index.js
Here is our example code:
window.addEventListener("message", (event) => {
const originsAllowed = [
'your Beacon Classic app URL'
];
if (originsAllowed.includes(event.origin)) {
switch (event.data.event) {
case 'beforeBeaconPageLoad':
console.log('beforeBeaconPageLoad Event data: ', event.data.data);
break;
case 'onBeaconPageLoad':
console.log('onBeaconPageLoad Event data: ', event.data.data);
break;
case 'loadedBeaconVideoMetadata':
console.log('loadedBeaconVideoMetadata Event data: ', event.data.data);
break;
case 'onBeaconPageChange':
console.log('onBeaconPageChange Event data: ', event.data.data);
break;
case 'onBeaconPageUnload':
console.log('onBeaconPageUnload Event data: ', event.data.data);
break;
}
}
},
false
);