Using the side panel
The side panel can be opened on the side <div>s of the player area. A chevron appears and the viewer can click the chevron to open and close the panel. The following video shows the behavior while displaying the event object when handling the onPlayerSidePanelDisplay
event:
Use cases
The following are appropriate use cases for the side panel:
- The side panel has been used for a chat application during live video.
- You can also have access to information about the video that is playing and could display information on the video in the panel.
Events
The following events can be used in your plugin code to control the side panel:
Event | Description |
---|---|
enablePlayerSidePanel |
Enables a button (using a chevron character) on the side of the Player area. This allows Beacon Classic app to show the side/hide panel when the viewer clicks the chevron. |
disablePlayerSidePanel |
Disables/hides the button (chevron character) on the side of the Player area. |
Dispatching event code syntax
When posting the enablePlayerSidePanel
and disablePlayerSidePanel
events no data need be passed. The syntax is:
window.postMessage({
event: 'enablePlayerSidePanel',
data: {}
}, window.location.origin);
Event listeners
The following events are listened for by the player when side panel events are dispatched in your OTT plugin code:
Event | Description |
---|---|
beforePlayerSidePanelDisplay |
Called before the animation to show the side panel starts. |
onPlayerSidePanelDisplay |
Called when the animation to show the side panel finishes. |
onPlayerSidePanelDisappear |
Called when the animation to hide the side panel finishes. |
Code syntax
The code for listening for the side panel events is the same as all other events.
window.addEventListener("message", (event) => {
switch (event.data.event) {
case 'onPlayerSidePanelDisplay':
populateSidePanel(event.data.data);
break;
}
});
The data contained in the event object pertinent to the side panel is as follows:
asset_id
: The Beacon Classic system video asset ID, which is different the video's Video Cloud ID.bc_account_id
: The Video Cloud account from which the video is taken.user_language
: The language code currently in use in the Beacon Classic app, taken from the Beacon API.video_id
: The Video Cloud ID of the video in the player.
An example event object when handling the onPlayerSidePanelDisplay
event:
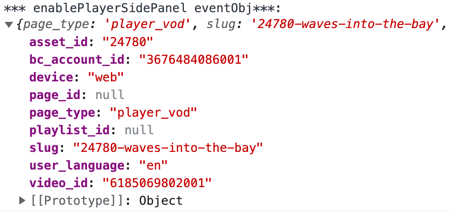
Module implementation
The following shows how functions using the side panel are implemented using JavaScript modules. Of course, you may implement your JavaScript in the manner of your choice. Further details on this module implementation can be found in the Implementing OTT Plugin Code Using Modules document.
The following code contains these functions. A brief descriptions is provided for each:
openSidePanel
: Dispatches theenablePlayerSidePanel
event so the open/hide chevron button is displayed in the player.enablePlayerSidePanel
: Places some example text in the panel. The key concept is locating thediv
element inside the panel using
var panelArea = document.getElementById('player_side_panel_hook');
clearSidePanel
: Clears the side panel of contents. Note that the viewer closing the side panel does NOT empty the contents, so functionality in this function can be useful.
const openSidePanel = () => {
window.postMessage({
event: 'enablePlayerSidePanel',
data: {}
}, window.location.origin);
};
const populateSidePanel = () => {
var panelArea = document.getElementById('player_side_panel_hook');
var content = '<h2>This is in the panel.</h2>');
panelArea.insertAdjacentHTML('afterbegin', content);
};
const clearSidePanel = () => {
var panelArea = document.getElementById('player_side_panel_hook');
panelArea.insertAdjacentHTML('afterbegin', ' ');
};
export { openSidePanel, populateSidePanel, clearSidePanel };